Table of Contents
In the ever-evolving landscape of cloud computing, Infrastructure as Code (IAC) has emerged as a cornerstone for managing and scaling complex cloud architectures. Two leading tools in this domain, Terraform and AWS CloudFormation, have simplified the deployment of cloud resources but cater to different use cases and preferences.
Whether you’re an IAC newcomer or looking to refine your skills, this article will unveil the 7 secrets to mastering IAC, with side-by-side comparisons of Terraform and CloudFormation. We’ll also include helpful code snippets to give you hands-on insights into how these tools operate, so let’s dive in!
#1: Understanding the Basics with Declarative Syntax
Both Terraform and CloudFormation employ declarative syntax, meaning you define the “what” of your infrastructure, and the tool figures out the “how.” Let’s look at a simple example of launching an Amazon S3 bucket in both tools.
Terraform Code to Create an S3 Bucket:
provider "aws" {
region = "us-east-1"
}
resource "aws_s3_bucket" "my_bucket" {
bucket = "my-terraform-bucket"
acl = "private"
}
CloudFormation Code to Create an S3 Bucket:
Resources:
MyS3Bucket:
Type: AWS::S3::Bucket
Properties:
BucketName: my-cloudformation-bucket
AccessControl: Private
In this example, notice the difference in file syntax. Terraform uses HCL (HashiCorp Configuration Language), while CloudFormation relies on YAML or JSON. If you’re newer to writing configurations, you might find HCL’s human-readable structure easier to grasp.
#2: Multi-Cloud vs AWS-Centric Focus
A critical aspect of mastering IAC lies in understanding which tool aligns better with your cloud strategy.
- Terraform: Ideal for managing multi-cloud environments or hybrid infrastructures. You can define resources for AWS, Azure, Google Cloud, Kubernetes, and more using a single configuration file.
For example, here’s a Terraform code snippet that deploys an Azure Resource Group alongside an AWS S3 Bucket:
# AWS provider
provider "aws" {
region = "us-east-1"
}
# Azure provider
provider "azurerm" {
features {}
}
# AWS S3 bucket
resource "aws_s3_bucket" "example_bucket" {
bucket = "example-terraform-bucket"
}
# Azure resource group
resource "azurerm_resource_group" "example_group" {
name = "example-resources"
location = "East US"
}
- CloudFormation: Limited to AWS resources, but it offers fine-grained control and advanced integration with AWS-native services like AWS Lambda, CloudWatch, and IAM policies.
#3: Managing Resource State
Managing resource state is another cornerstone of IAC mastery. Terraform relies on state files, whereas CloudFormation uses AWS-managed stacks.
Terraform State Management Example:
# Commands to manage Terraform state
terraform init # Initialize your directory
terraform plan # Preview changes to your infrastructure
terraform apply # Apply the changes
terraform show # View the current state
Terraform stores the state file locally or remotely (e.g., in S3 or HashiCorp Vault), ensuring precise management of resources across iterations.
CloudFormation State Management:
CloudFormation automatically manages resource states via StackSets. For example, you can view the status of your stack by navigating to the AWS Management Console or running:
aws cloudformation describe-stacks --stack-name my-stack
Terraform requires more manual oversight of its state, offering greater flexibility, whereas CloudFormation abstracts state management for simplicity.
#4: Modularization of Infrastructure
When working on large-scale deployments, modularity is key to reusability and cleaner configurations. Let’s explore how modularization works in Terraform and CloudFormation.
Terraform Module Example:
You can create a reusable module for an AWS VPC:
# Module usage in main Terraform configuration
module "vpc" {
source = "./modules/vpc"
cidr_block = "10.0.0.0/16"
enable_dns_support = true
}
The source
parameter points to a separate folder containing the VPC definitions, making your main infrastructure file concise.
CloudFormation Nested Stack Example:
In CloudFormation, you can create nested stacks for modularity:
Resources:
VPCStack:
Type: AWS::CloudFormation::Stack
Properties:
TemplateURL: https://mybucket.s3.amazonaws.com/vpc-template.yaml
With both tools, modularization reduces redundancy and simplifies large configurations, but Terraform’s approach is widely considered more developer-friendly.
#5: Testing and Validation
Validating your configurations can save hours of debugging time, and both Terraform and CloudFormation provide mechanisms to test your code.
Terraform Plan for Validation:
terraform validate # Validates the configuration file
terraform plan # Simulates changes
Terraform’s plan
command explicitly shows the actions Terraform will perform, offering confidence before any changes.
CloudFormation Validation Example:
aws cloudformation validate-template --template-body file://template.yaml
CloudFormation’s validation ensures syntax correctness, but it lacks a preview feature akin to Terraform’s plan.
#6: Error Handling is Key
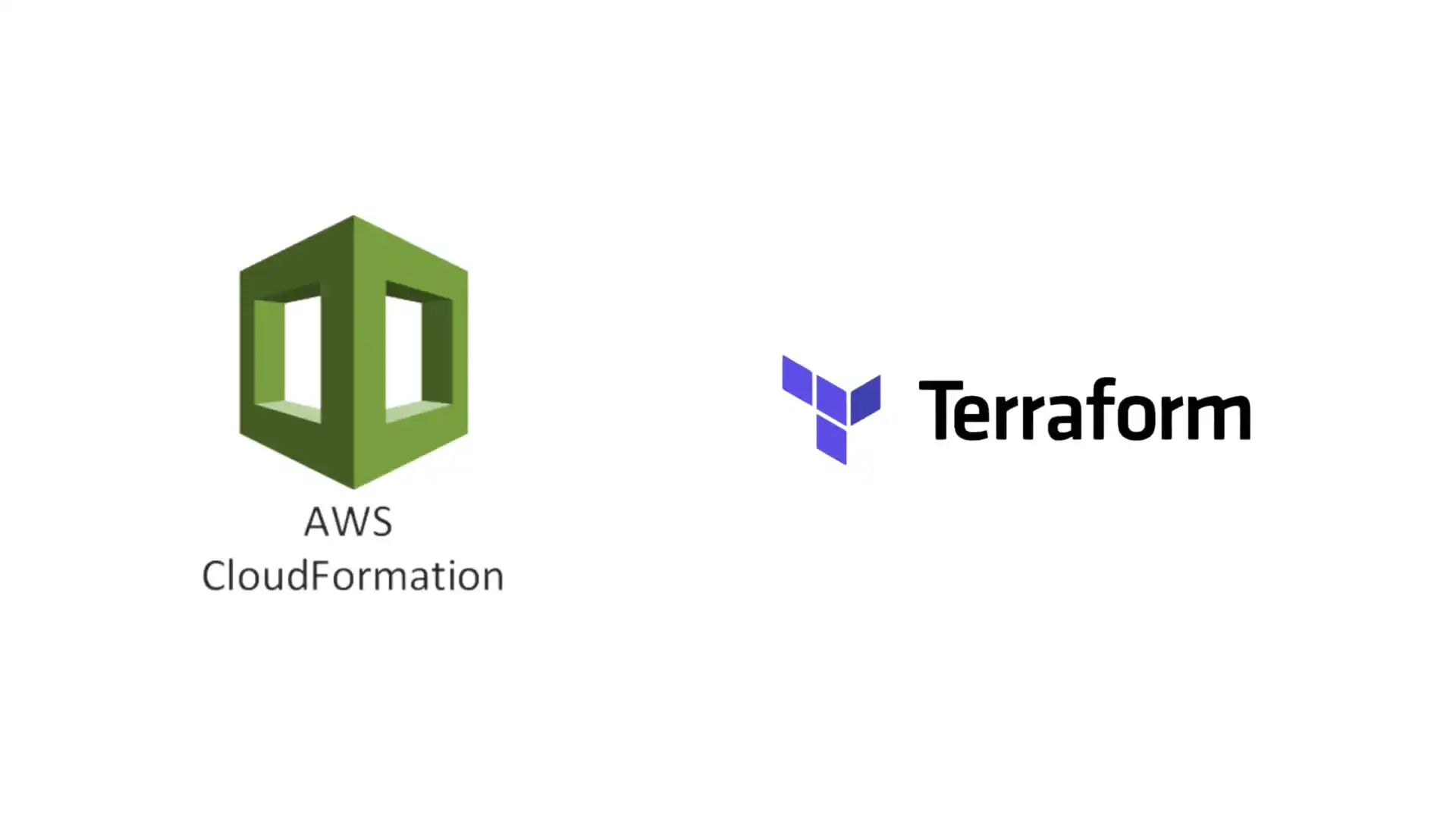
Error handling is inevitable in IAC operations, but how you address errors can significantly impact efficiency.
- Terraform: Errors are generally displayed in the CLI during the
terraform apply
phase, making troubleshooting straightforward. Failed plans do not implement changes, safeguarding your existing infrastructure. - CloudFormation: Errors are logged in AWS CloudTrail and CloudWatch. Here is how you can view stack events to pinpoint issues:
aws cloudformation describe-stack-events --stack-name my-stack
Effective error handling requires familiarity with these tools’ log mechanisms—Terraform leans on direct feedback, while CloudFormation relies heavily on AWS’s broader monitoring services.
#7: Infrastructure Drift Detection
Detecting infrastructure drift is critical for ensuring that your deployed resources match their intended configurations.
Terraform Drift Detection:
Terraform identifies drift during the terraform plan
process. Here’s a quick look:
terraform plan # Detects differences between state and actual infrastructure
CloudFormation Drift Detection:
CloudFormation offers a drift detection feature explicitly for this purpose:
aws cloudformation detect-stack-drift --stack-name my-stack
Once drift is detected, CloudFormation outputs the mismatched resources for you to address.
Conclusion
Choosing between Terraform and CloudFormation depends on your cloud strategy, expertise, and operational requirements.
- For multi-cloud and portability, Terraform offers unmatched flexibility and a robust modular system.
- If you’re deeply integrated with AWS, CloudFormation simplifies resource creation with tightly coupled AWS-native solutions.
By understanding these 7 secrets—key features, portability, state management, modularity, validation, error handling, and drift detection—you’ll empower yourself to master Infrastructure as Code with either Terraform or CloudFormation.
Start small, experiment with the code snippets provided here, and gradually scale your skills as you explore advanced configurations. Remember, the best tool for the job is the one that aligns with your specific needs!