Table of Contents
Effective state management in React is essential for building scalable and maintainable applications.
In this article, we’ll explore three powerful tools for state management: Redux, Zustand, and the Context API.
Learn their features, use cases, and when to use each for managing state effectively .
Understanding State Management in React
In React, state represents the dynamic parts of your application—such as user inputs, UI toggles, and fetched data. While React’s local state (using useState
) works for small-scale apps, larger applications often require a centralized state management solution to:
- Share data across deeply nested components.
- Avoid prop-drilling.
- Improve maintainability and scalability.
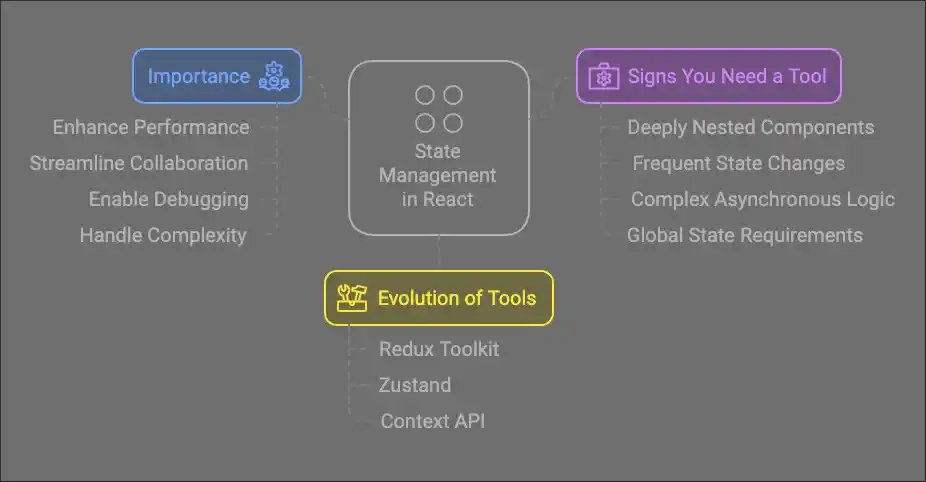
Why State Management Important?
State management ensures consistency and predictability in your application’s behavior. By using a robust state management tool, developers can:
- Enhance Performance: Optimize re-renders and minimize unnecessary computations.
- Streamline Collaboration: Maintain clear and understandable state structures for teams.
- Enable Debugging: Track state changes effectively for faster issue resolution.
- Handle Complexity: Manage interconnected components and asynchronous data flows seamlessly.
Signs You Need a State Management Tool
If your React application exhibits any of the following characteristics, it may be time to consider a state management library:
- Deeply Nested Components: Passing props through multiple layers becomes unwieldy.
- Frequent State Changes: Managing and syncing state across components leads to bugs.
- Complex Asynchronous Logic: Handling API calls and side effects becomes cumbersome.
- Global State Requirements: Data needs to be accessible across various parts of the application.
The Evolution of State Management in React
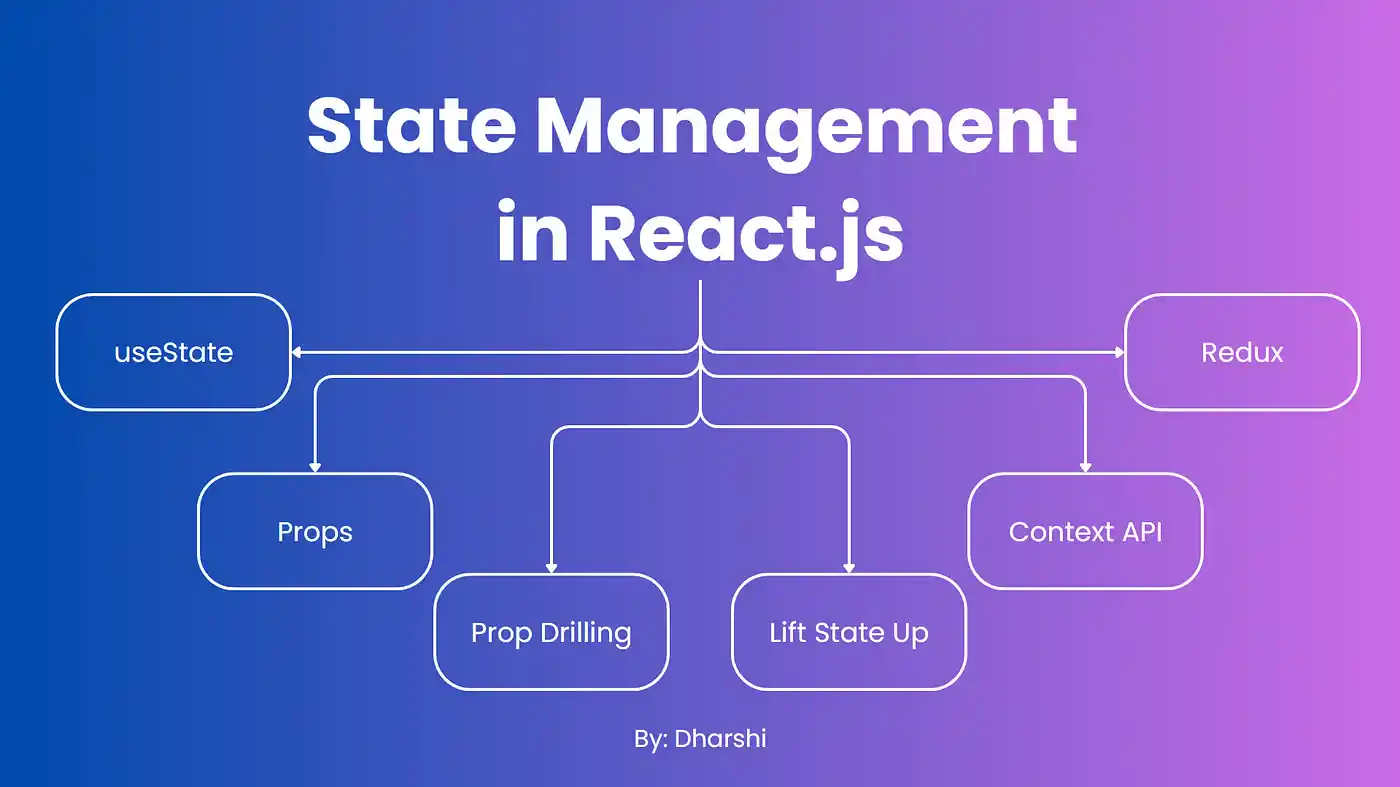
React’s ecosystem has evolved to include powerful state management tools like Redux Toolkit, Zustand, and Context API. Each offers unique advantages tailored to different project needs. In this article, we’ll explore these tools to help you choose the right one for your next React project.
1. Redux Toolkit: The Modern Powerhouse for State Management in React
Redux Toolkit (RTK) is the officially recommended way to use Redux. It simplifies many aspects of Redux, such as configuration, boilerplate reduction, and state immutability. By addressing common pain points, RTK makes state management in React more efficient and developer-friendly.
Core Principles of Redux Toolkit:
- Single Source of Truth: Similar to Redux, RTK maintains a single JavaScript object for the state.
- Immutable State Updates: RTK uses Immer.js under the hood to simplify immutable state updates.
- Simplified Syntax: With pre-configured features, RTK reduces the amount of code you need to write.
Key Features of Redux Toolkit:
- Integrated DevTools: RTK seamlessly integrates with Redux DevTools, making debugging easy.
- Simplified Configuration: The
configureStore
method sets up the store with sensible defaults. - Built-in Middleware: RTK includes middleware for handling asynchronous logic, like
createAsyncThunk
. - Slice Management: The
createSlice
API simplifies defining reducers and actions in one place. - TypeScript Support: RTK offers excellent TypeScript support, making type-safe state management a breeze.
When to Use Redux Toolkit:
- Applications with complex state management needs involving multiple components.
- Projects requiring advanced debugging, logging, or middleware capabilities.
- Scenarios where a centralized state store provides clarity and maintainability.
- Large teams collaborating on sophisticated applications.
Example Use Case:
In an e-commerce application, Redux Toolkit can manage product data, user authentication, and shopping cart states efficiently. The createAsyncThunk
method simplifies handling API calls, while slices modularize the state.
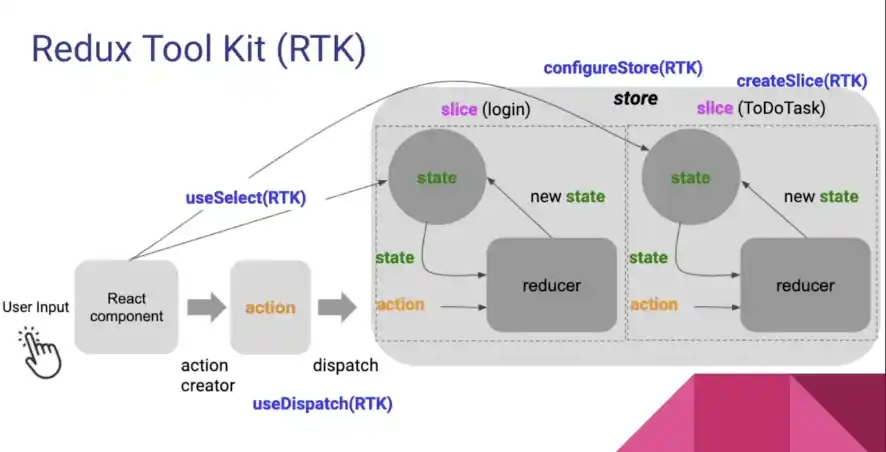
Example:
import { createStore } from 'redux';
const initialState = { count: 0 };
function counterReducer(state = initialState, action) {
switch (action.type) {
case 'INCREMENT':
return { count: state.count + 1 };
case 'DECREMENT':
return { count: state.count - 1 };
default:
return state;
}
}
const store = createStore(counterReducer);
2. Zustand: Lightweight and Flexible State Management for React
Zustand (German for “state”) is a lightweight, flexible library for state management. It offers a modern alternative to Redux for applications that prioritise simplicity and speed.
Core Principles of Zustand:
- No Boilerplate: Zustand avoids the complexity of reducers and actions.
- State Without Context: It doesn’t rely on React’s Context API, which improves performance.
- Direct State Updates: Zustand allows you to update state directly through JavaScript functions.
Key Features of Zustand:
- Global Store Without Context: Zustand maintains a global state without the performance overhead of React’s Context API.
- Minimalistic API: Its intuitive API focuses on simplicity and ease of use.
- Optimized Performance: Zustand’s architecture ensures that only the necessary components re-render.
- Middleware Integration: Supports middleware for actions like logging or persistence.
Advanced Benefits of Zustand
1. Flexibility for Any Application
From small-scale projects to more complex applications, Zustand’s scalability and simplicity make it a versatile choice. Whether you’re building a personal blog, a real-time chat app, or an e-commerce platform, Zustand adapts to your needs without introducing unnecessary complexity.
2. Developer-Friendly Design
Zustand’s approach aligns closely with modern development practices. Its reliance on plain JavaScript and direct manipulation makes it easy to onboard new team members and maintain codebases over time.
3. Selective Re-Renders
Zustand’s ability to trigger updates only in components that depend on specific state slices is invaluable for performance optimization, particularly in apps with dynamic and high-frequency updates.
4. Unopinionated and Modular
Zustand is unopinionated, giving you the freedom to structure your state management the way you prefer. Its modular nature allows seamless integration with other libraries and tools in your tech stack.
When to Use Zustand:
- Projects prioritizing simplicity and minimal overhead.
- Applications with dynamic or flexible state updates that don’t fit Redux’s strict patterns.
- Small-to-medium apps where the complexity of Redux would be overkill.
- Scenarios requiring selective re-renders for performance optimization.
Why Developers Love Zustand
- Ease of Use: Its minimalistic API eliminates the learning curve associated with traditional state management libraries.
- Performance: Zustand’s innovative design delivers faster apps with fewer re-renders.
- Flexibility: From prototyping to production, Zustand scales with your project requirements.
- Community Support: A growing community of developers ensures regular updates and valuable resources.
Example Use Case:
A blogging platform can use Zustand to manage the state of drafts, user preferences, and theme settings. Zustand’s flexible API allows straightforward implementation without complex boilerplate.
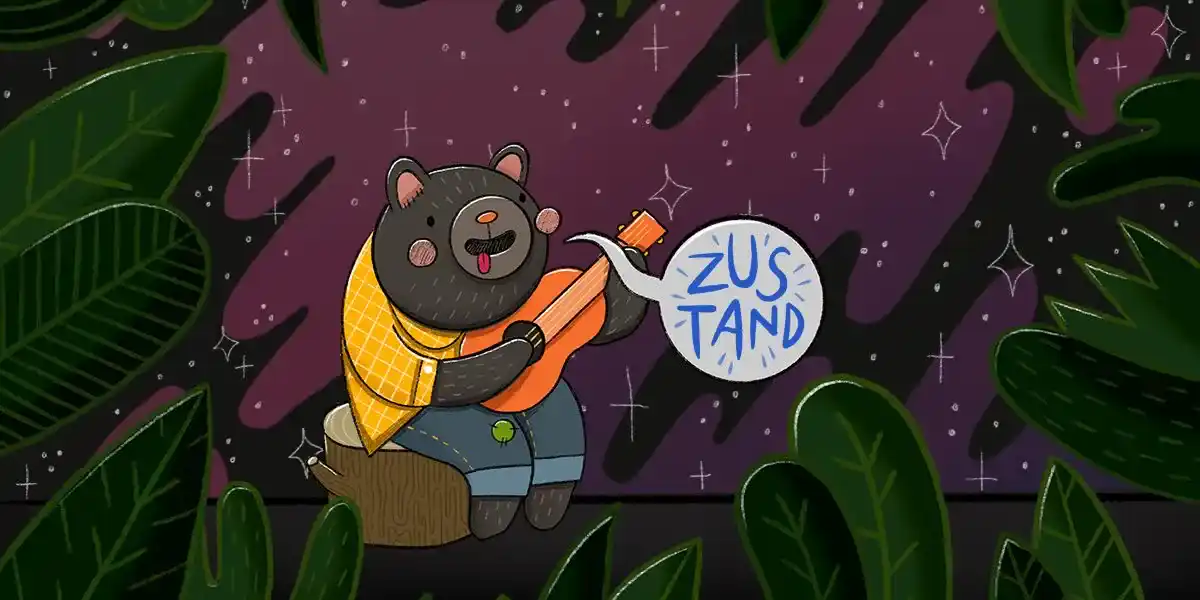
Example:
import create from 'zustand';
const useStore = create((set) => ({
count: 0,
increment: () => set((state) => ({ count: state.count + 1 })),
decrement: () => set((state) => ({ count: state.count - 1 })),
}));
function Counter() {
const { count, increment, decrement } = useStore();
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
);
}
3. Context API: Built-In and Powerful State Management for React
The Context API is a native React feature for managing state across components without prop drilling. It’s ideal for simple or localized state management scenarios.
Core Principles of Context API:
- Data Sharing: Provides a way to share state between components without passing props explicitly.
- Subscription Mechanism: Components subscribe to changes in the context value and update automatically.
- Native to React: No external dependencies or libraries required.
Key Features of Context API:
- Lightweight Solution: Perfect for managing static or simple global states.
- Flexible Usage: Works seamlessly for small apps or specific state slices.
- Integration-Friendly: Can be combined with other libraries like Redux or Zustand for hybrid state management.
When to Use Context API:
- Applications with simple, static global states such as theme toggling or language preferences.
- Projects aiming to avoid adding external dependencies.
- Scenarios requiring isolated state management within a component tree.
Limitations of Context API:
- Performance Concerns: Frequent updates to large contexts can cause unnecessary re-renders.
- Not Scalable: Better suited for small-scale state management; larger applications may require Redux or Zustand.
Example Use Case:
A weather application can use Context API to manage and share user preferences, like temperature units (Celsius or Fahrenheit), across components.
Example:
import React, { createContext, useContext, useState } from 'react';
const CountContext = createContext();
function CountProvider({ children }) {
const [count, setCount] = useState(0);
return (
<CountContext.Provider value={{ count, setCount }}>
{children}
</CountContext.Provider>
);
}
function Counter() {
const { count, setCount } = useContext(CountContext);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
<button onClick={() => setCount(count - 1)}>Decrement</button>
</div>
);
}
function App() {
return (
<CountProvider>
<Counter />
</CountProvider>
);
}
Choosing the Right Solution for Effective State Management in React
The choice between Redux, Zustand, and Context API depends on your application’s complexity, team size, and project goals. Here’s a quick guide:
Comparing Redux Toolkit, Zustand, and Context API
Feature | Redux Toolkit | Zustand | Context API |
---|---|---|---|
Ease of Use | Moderate, with pre-configured APIs | Very easy, minimal setup | Easy, built into React |
Performance | High, but depends on implementation | High, optimized re-renders | Can suffer with large updates |
Boilerplate | Minimal compared to Redux | Minimal | None |
Scalability | Highly scalable | Scalable for medium apps | Limited |
Async Handling | Built-in with createAsyncThunk | Requires manual setup | Not designed for async tasks |
DevTools Support | Yes, integrated | Limited | None |
Each solution has its strengths and is suited to different project requirements. Choose the one that aligns best with your app’s complexity, team size, and performance needs.
Conclusion
Effective state management in React is crucial for building robust and scalable applications. The right choice depends on the project’s requirements and the team’s preferences:
Why Choose Redux Toolkit?
- For large-scale applications with intricate state logic.
- When your team needs advanced tools like middleware and DevTools for debugging.
- Ideal for collaborative projects with multiple developers.
Why Choose Zustand?
- Perfect for projects prioritizing simplicity and minimal configuration.
- Suitable for small-to-medium apps where Redux might feel too heavy.
- Ideal for apps requiring dynamic state updates without strict patterns.
Why Choose Context API?
- Best for small, simple projects or isolated state management needs.
- Works well when avoiding additional dependencies is a priority.
- Ideal for specific state slices, like user settings or theme toggling.
By understanding the strengths and limitations of each solution, you can make an informed decision tailored to your app’s needs. Whether you’re managing global state for a complex enterprise app or keeping it simple for a personal project, these tools empower you to build efficient and maintainable React applications.