Table of Contents
When you hear “SOLID principles,” do you think of a rock band or maybe the feeling of relief after completing a big meal? Nope, SOLID principles are actually a set of design principles that can turn your Laravel code into a masterpiece (or at least stop it from looking like spaghetti).
Let’s dive into SOLID principles and learn how to implement them in Laravel — all while having a bit of fun!
What Are SOLID Principles?
SOLID is an acronym for five principles that make software designs easier to understand, extend, and maintain. Think of it as the Avengers of programming principles: each hero (principle) is strong on their own, but together, they save your code from chaos.
Here’s what SOLID stands for:
- Single Responsibility Principle (SRP)
- Open/Closed Principle (OCP)
- Liskov Substitution Principle (LSP)
- Interface Segregation Principle (ISP)
- Dependency Inversion Principle (DIP)
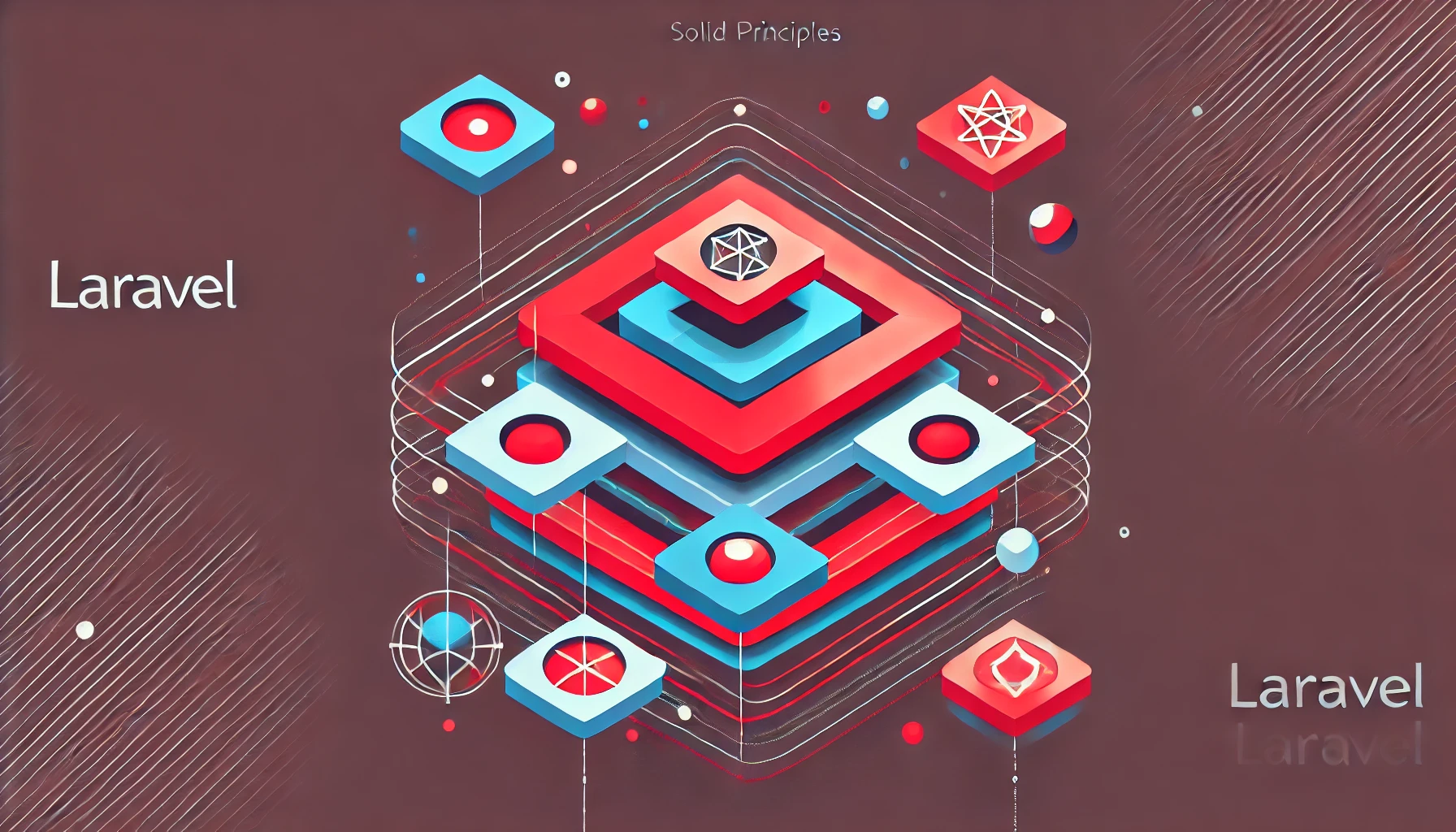
Single Responsibility Principle (SRP)
“One class, one job. Period.”
Imagine your manager gives you a to-do list with 50 items. Overwhelming, right? Classes feel the same way when you dump multiple responsibilities on them. The Single Responsibility Principle says a class should have only one reason to change.
Example
A controller is NOT your dumping ground for business logic, database queries, or secret cookie recipes. Let’s clean it up:
Bad Example
class OrderController extends Controller
{
public function store(Request $request)
{
$order = new Order();
$order->user_id = $request->user()->id;
$order->total = $this->calculateTotal($request->items);
$order->save();
$this->sendOrderEmail($order);
return response()->json(['success' => true]);
}
private function calculateTotal($items)
{
// Calculating total...
}
private function sendOrderEmail($order)
{
// Sending email...
}
}
Good Example
Break this into separate responsibilities:
class OrderController extends Controller
{
public function store(Request $request, OrderService $orderService)
{
$orderService->createOrder($request);
return response()->json(['success' => true]);
}
}
class OrderService
{
public function createOrder($request)
{
$order = new Order();
$order->user_id = $request->user()->id;
$order->total = $this->calculateTotal($request->items);
$order->save();
$this->sendOrderEmail($order);
}
private function calculateTotal($items)
{
// Calculating total...
}
private function sendOrderEmail($order)
{
// Sending email...
}
}
Why? If the email-sending logic changes, you touch OrderService
, not OrderController
. Responsibility: clear. Code: happy.
Open/Closed Principle (OCP)
“Open for extension, closed for modification.”
Think of OCP as that no-touch sign at a museum: extend functionality without breaking or modifying existing code.
Example
Let’s say we’re calculating discounts.
Bad Example
class DiscountCalculator
{
public function calculate($order)
{
if ($order->user->isVip()) {
return $order->total * 0.2; // VIP discount
}
if ($order->total > 100) {
return $order->total * 0.1; // Bulk discount
}
return 0;
}
}
What happens if tomorrow you introduce a “Black Friday” discount? Boom — modification chaos!
Good Example
Use polymorphism:
interface Discount
{
public function calculate($order);
}
class VipDiscount implements Discount
{
public function calculate($order)
{
return $order->user->isVip() ? $order->total * 0.2 : 0;
}
}
class BulkDiscount implements Discount
{
public function calculate($order)
{
return $order->total > 100 ? $order->total * 0.1 : 0;
}
}
You can now extend this without modifying the original classes. Add a BlackFridayDiscount
or even a PizzaLoverDiscount
!
Liskov Substitution Principle (LSP)
“Derived classes must be substitutable for their base classes.”
If your code screams in pain when you replace a parent class with a child class, you’ve broken LSP. This principle ensures subclasses behave as expected without surprises.
Example
Let’s take an example of birds :
Bad Example
class Bird
{
public function fly()
{
return "I’m flying!";
}
}
class Penguin extends Bird
{
public function fly()
{
throw new Exception("Penguins can’t fly!");
}
}
A flying penguin? Sounds like a new Marvel character.
Good Example
Separate behaviors appropriately:
interface Bird
{
public function move();
}
class Sparrow implements Bird
{
public function move()
{
return "I’m flying!";
}
}
class Penguin implements Bird
{
public function move()
{
return "I’m swimming!";
}
}
Now your code won’t crash at the zoo.
Interface Segregation Principle (ISP)
“Don’t force classes to implement what they don’t use.”
Imagine ordering a pizza but being forced to learn the entire menu. Interfaces feel the same way when overloaded.
Example
Let’s take an example :
Bad Example
interface Payment
{
public function payWithCreditCard();
public function payWithPaypal();
public function payWithBitcoin();
}
class CreditCardPayment implements Payment
{
public function payWithCreditCard()
{
// Process credit card payment
}
public function payWithPaypal()
{
throw new Exception("Not implemented.");
}
public function payWithBitcoin()
{
throw new Exception("Not implemented.");
}
}
A payment interface that only works for one method? That’s like bringing a spoon to a knife fight.
Good Example
Split the interface into smaller, specific contracts:
interface CreditCardPayment
{
public function payWithCreditCard();
}
interface PaypalPayment
{
public function payWithPaypal();
}
interface BitcoinPayment
{
public function payWithBitcoin();
}
Now, you only implement what you need.
Dependency Inversion Principle (DIP)
“Depend on abstractions, not concretions.”
Think of this as having your coffee brewed by a barista, not depending on a specific brand of beans. The principle promotes flexibility by relying on abstractions.
Example
Let’s take an example :
Bad Example
class Notification
{
public function sendEmail($message)
{
// Email sending logic...
}
}
If tomorrow you need to send SMS instead of email, you’ll cry (and refactor).
Good Example
interface Notifier
{
public function send($message);
}
class EmailNotifier implements Notifier
{
public function send($message)
{
// Send email
}
}
class SMSNotifier implements Notifier
{
public function send($message)
{
// Send SMS
}
}
class NotificationService
{
private $notifier;
public function __construct(Notifier $notifier)
{
$this->notifier = $notifier;
}
public function notify($message)
{
$this->notifier->send($message);
}
}
Now, swapping EmailNotifier
with SMSNotifier
is as easy as switching coffee brands.
Wrapping Up with Laravel Flavor
SOLID principles might sound intimidating at first, but they’re your secret weapon for writing clean, maintainable code. Remember:
- SRP = Fewer jobs per class = Happier code.
- OCP = Add, don’t edit.
- LSP = Respect substitution rules.
- ISP = Smaller interfaces = Easier implementation.
- DIP = Abstract dependencies = Flexible designs.
So go ahead, sprinkle these principles into your Laravel projects. Your future self (and your team) will thank you. And remember: keep it SOLID, not spaghetti!
Conclusion
Embracing the SOLID principles in your Laravel projects isn’t just about writing cleaner code — it’s about creating systems that are easier to maintain, extend, and love. By giving each class a clear purpose, keeping your code flexible, and relying on abstractions, you’re setting yourself up for a more enjoyable development experience (and fewer late-night debugging sessions).
Think of these principles as your coding gym: they might feel like a workout at first, but the gains — maintainable, scalable, and robust applications — are absolutely worth it. Whether you’re tackling a personal project or building the next big thing, a SOLID foundation ensures your code stands the test of time (and feature requests).
So next time your code starts resembling spaghetti, remember these principles and ask yourself, “Am I keeping it SOLID?” With Laravel by your side and these principles in your toolkit, you’re unstoppable. Now go forth and write some legendary code!
READ MORE:
Web Application Development: A Comprehensive Guide For 2024
Top Emerging Application Modernization Trends for 2024