Table of Contents
Master the Art of Effortlessly Accessing Public Method in React Functional Components
React functional components have become the standard choice for building React applications due to their simplicity, performance, and the introduction of hooks. One common challenge developers face is accessing methods or functionality within a functional component from its parent component. While class components provided this capability through instance methods, functional components require a slightly different approach. Here’s how you can achieve this effectively and efficiently.
This article will cover how to access or invoke “Public Method in react” of a functional component from its parent. We will explore two primary approaches:
- Using useImperativeHandle and
forwardRef
to expose methods in the child component. - Using callback functions passed from the parent to the child component.
Let’s dive into each approach with examples!
Exposing Public Method in React Using useImperativeHandle and forwardRef
To expose Public Method in react methods in a functional component for the parent to call, you can use the useImperativeHandle hook combined with forwardRef. This pattern enables you to define methods in the child component and make them accessible to the parent via a ref.
How It Works
- forwardRef allows the parent component to pass a ref to the child.
- useImperativeHandle lets you specify which methods or values should be accessible via the ref.
Example
ChildComponenet.jsx
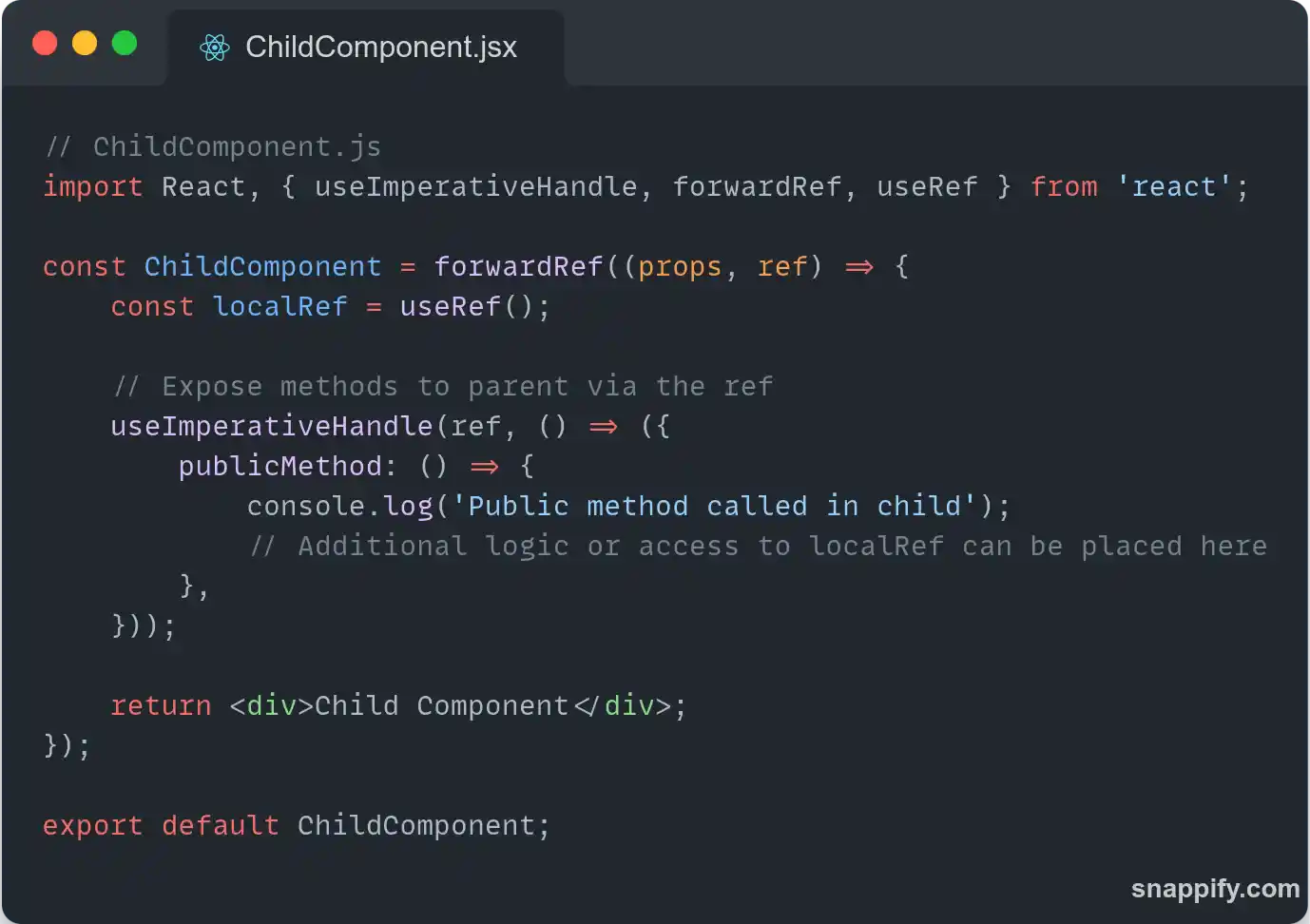
In this example, the ChildComponent
exposes a publicMethod
via the ref. Now let’s see how the parent component can call this method.
ParentComponent.jsx
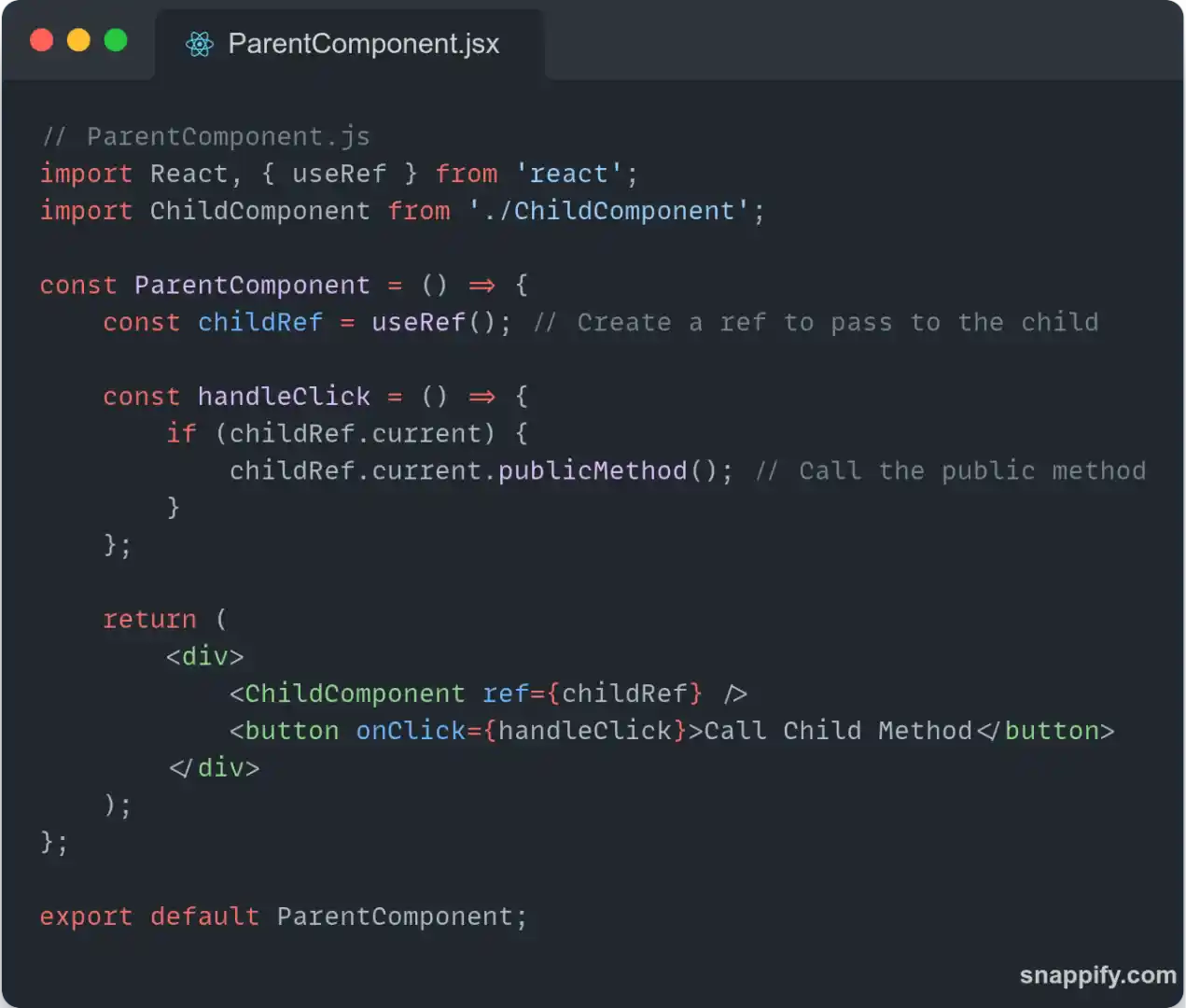
In this example, the ParentComponent holds a reference (ref
) to the ChildComponent and uses it to call the publicMethod
exposed by the child. When the button in the parent is clicked, the handleClick
function triggers this method, enabling seamless parent-child interaction.
Key Points:
- The parent component holds a ref(childref) to the ChildComponenet.
- When the button in the parent is clicked, it triggers the handleClick function, which calls the
publicMethod
of the child. - The child component exposes the publicMethod using useImperativeHandle, making it available to the parent.
This approach allows you to access the child’s internal functionality from outside, making it behave similarly to how class components expose public method in react.
Summary
Accessing public method in React functional components from parent components is an advanced technique that enhances the communication and interaction between components. Functional components, unlike class components, do not inherently support public methods because they are purely functions. However, React offers powerful tools like forwardRef and useImperativeHandle to enable this functionality.
forwardRef is a higher-order component that allows you to pass a reference from a parent component to a child component. This reference can be used to directly access the child’s internal methods or properties. However, simply forwarding a reference does not define which methods can be accessed. This is where useImperativeHandle comes into play.
useImperativeHandle works in conjunction with forwardRef to customize the methods or properties that the child exposes to the parent. By using this hook, you can control what the parent component can access, ensuring a clean and well-encapsulated design. This approach is particularly useful in scenarios like resetting forms, controlling modal states, triggering custom animations, or other cases where the parent needs fine-grained control over the child.
To use this technique:
- Wrap the child component with forwardRef.
- In the child, use useImperativeHandle to define the methods you want to expose.
- In the parent, create a reference using
useRef
and pass it to the child component via theref
prop. - The parent can then invoke the exposed methods using the reference.
This pattern provides a structured and modular way to enable parent-child interactions without compromising the reusability or simplicity of the child component. It helps developers manage complex behaviors efficiently while adhering to React’s principles of unidirectional data flow and component encapsulation.